ImageView in Andorid
In this tutorial we will see how to display image with help of ImageView.
we will load image on Button Click from drawable resouece folder in app .
Lets get started.
1. open android studio
2. create a new project
3. Set application name ImageView
4. I used package path as com.androidcodr.imageview click next.
5. On this screen set minimum sdk API 16 click next.
6. Add empty activity. click next.
7. keep Activity name as MainActivity click finish. wait till project load completely.
8. Goto activity_main.xml
9. if you are having constrainLayout change it to RelativeLayout.
Just replace android.support.constraint.ConstraintLayout with RelativeLayout
10. change Hello world textview property as follows
<TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/textView" android:text="Click button to change Image" />
11. now drag n drop button from palette below the textview.
12. on button click we will call method to change imagae so set onClick property as
android:onClick=”changeimg”
<Button android:text="Change" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/textView" android:layout_centerHorizontal="true" android:id="@+id/button" android:onClick="changeimg" />
13. now drag n drop ImageView from palette below the button.
this will ask you to set imagae immediately
so select ic_launcher image from drawable folder.
<ImageView android:layout_width="match_parent" android:layout_height="match_parent" app:srcCompat="@mipmap/ic_launcher" android:layout_below="@+id/button" android:layout_centerHorizontal="true" android:id="@+id/imageView" />
There are different scale type property for imageView

CENTER : Displays the image centered in the view with no scaling.

CENTER_CROP : Scales the image such that both the x and y dimensions are greater than or equal to the view, while maintaining the image aspect ratio; centers the image in the view

CENTER_INSIDE : Scales the image to fit inside the view, while maintaining the image aspect ratio. If the image is already smaller than the view, then this is the same as center
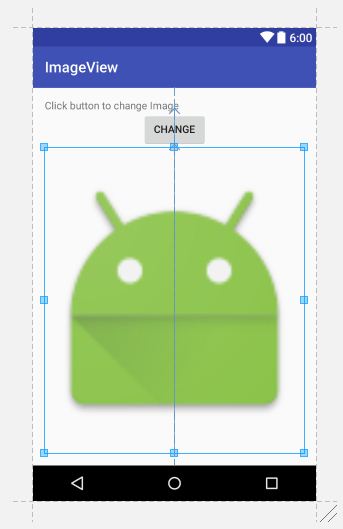
FIT_CENTER : Scales the image to fit inside the view, while maintaining the image aspect ratio. At least one axis will exactly match the view, and the result is centered inside the view

FIT_START : Same as fitCenter but aligned to the top left of the view
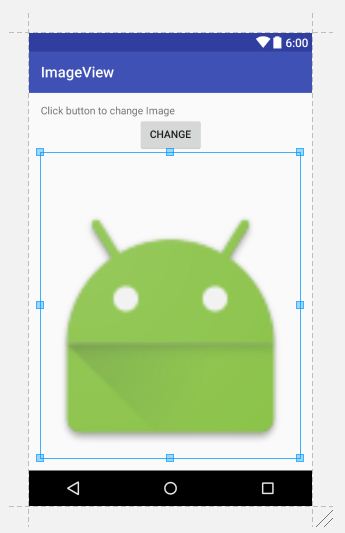
FIT_END : Same as fitCenter but aligned to the bottom right of the view
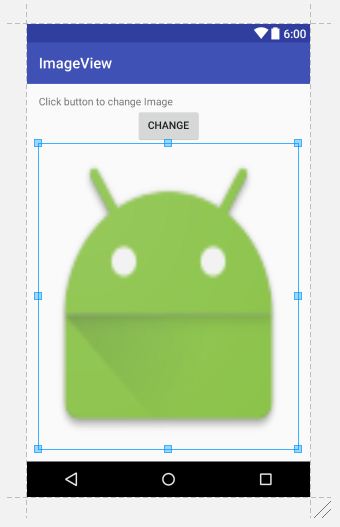
FIT_XY : Scales the x and y dimensions to exactly match the view size. dDoes not maintain the image aspect ratio

MATRIX : Scales the image using a supplied Matrix class. The matrix can be supplied using the setImageMatrix method. A Matrix class can be used to apply transformations such as rotations to an image
14. now we need one more image which will appear or load on ImageView
when button is clicked.
so androidcodrlogo.jpg
copy this image and paste in drawable folder.
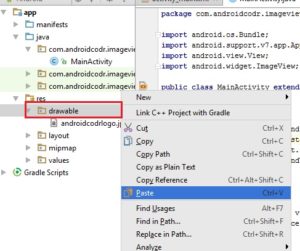
15. go to MainActivity.java
Declare ImageView
ImageView dpimage;
Initialize in onCreate method
dpimage=(ImageView) findViewById(R.id.imageView);
16. now add method changeimg for button click as follows
public void changeimg(View view){ }
17. set image for imageview
use setImageResource method from ImageView class.
pass image resource id.
dpimage.setImageResource(R.drawable.androidcodrlogo);
18. compile and build app.
19. run app

20. we can see default image loaded
21. now click button change
22. now image is changed our logo image is shown.
Here full source of activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.androidcodr.imageview.MainActivity"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/textView" android:text="Click button to change Image" /> <Button android:text="Change" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/textView" android:layout_centerHorizontal="true" android:id="@+id/button" android:onClick="changeimg" /> <ImageView android:layout_width="match_parent" android:layout_height="match_parent" app:srcCompat="@mipmap/ic_launcher" android:layout_below="@+id/button" android:layout_centerHorizontal="true" android:id="@+id/imageView" /> </RelativeLayout>
Here is full source of MainActivity.java
package com.androidcodr.imageview; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.ImageView; public class MainActivity extends AppCompatActivity { ImageView dpimage; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); dpimage=(ImageView) findViewById(R.id.imageView); } public void changeimg(View view){ dpimage.setImageResource(R.drawable.androidcodrlogo); } }
Thanks.
If you Like post share with your Friends.
Subscribe us.
Follow us on Facebook Twitter and Google+